使用 DALL-E 生成和编辑图像
此笔记本展示了如何使用 OpenAI 的 DALL-E 图像 API 端点。
共有三个 API 端点:
- Generations:根据输入的标题生成一个或多个图像
- Edits:编辑或扩展现有图像
- Variations:生成输入图像的变体
设置
- 导入你需要的包
- 导入您的 OpenAI API 密钥
- 设置保存图片的目录
# imports import openai # OpenAI Python library to make API calls import requests # used to download images import os # used to access filepaths from PIL import Image # used to print and edit images # set API key openai.api_key = os.environ.get("OPENAI_API_KEY")
# set a directory to save DALL-E images to image_dir_name = "images" image_dir = os.path.join(os.curdir, image_dir_name) # create the directory if it doesn't yet exist if not os.path.isdir(image_dir): os.mkdir(image_dir) # print the directory to save to print(f"{image_dir=}")
image_dir='./images'
Generations
生成 API 端点根据文本提示创建图像。
所需输入:
- prompt(str):所需图像的文本描述。 最大长度为 1000 个字符。
可选输入:
- n (int):要生成的图像数。 必须介于 1 和 10 之间。默认为 1。
- size (str):生成图像的大小。 必须是“256×256”、“512×512”或“1024×1024”之一。 较小的图像速度更快。 默认为“1024×1024”。
- response_format (str):返回生成图像的格式。 必须是“url”或“b64_json”之一。 默认为“网址”。
- user (str):代表您的最终用户的唯一标识符,这将有助于 OpenAI 监控和检测滥用行为。 了解更多。
# create an image # set the prompt prompt = "A cyberpunk monkey hacker dreaming of a beautiful bunch of bananas, digital art" # call the OpenAI API generation_response = openai.Image.create( prompt=prompt, n=1, size="1024x1024", response_format="url", ) # print response print(generation_response)
{ "created": 1667611641, "data": [ { "url": "https://oaidalleapiprodscus.blob.core.windows.net/private/org-l89177bnhkme4a44292n5r3j/user-dS3DiwfhpogyYlat6i42W0QF/img-SFJhix3AV4bmPFvqYRJDkssp.png?st=2022-11-05T00%3A27%3A21Z&se=2022-11-05T02%3A27%3A21Z&sp=r&sv=2021-08-06&sr=b&rscd=inline&rsct=image/png&skoid=6aaadede-4fb3-4698-a8f6-684d7786b067&sktid=a48cca56-e6da-484e-a814-9c849652bcb3&skt=2022-11-05T01%3A27%3A21Z&ske=2022-11-06T01%3A27%3A21Z&sks=b&skv=2021-08-06&sig=0ZHl38v5UTFjA7V5Oshu8M58uHI5itEfvo2PX0aO6kA%3D" } ] }
注意:如果您收到此错误 – AttributeError: module 'openai' has no attribute 'Image'
– 您需要将 OpenAI 包升级到最新版本。 您可以通过在终端中运行 pip install openai --upgrade
来完成此操作。
# save the image generated_image_name = "generated_image.png" # any name you like; the filetype should be .png generated_image_filepath = os.path.join(image_dir, generated_image_name) generated_image_url = generation_response["data"][0]["url"] # extract image URL from response generated_image = requests.get(generated_image_url).content # download the image with open(generated_image_filepath, "wb") as image_file: image_file.write(generated_image) # write the image to the file
# print the image print(generated_image_filepath) display(Image.open(generated_image_filepath))
./images/generated_image.png
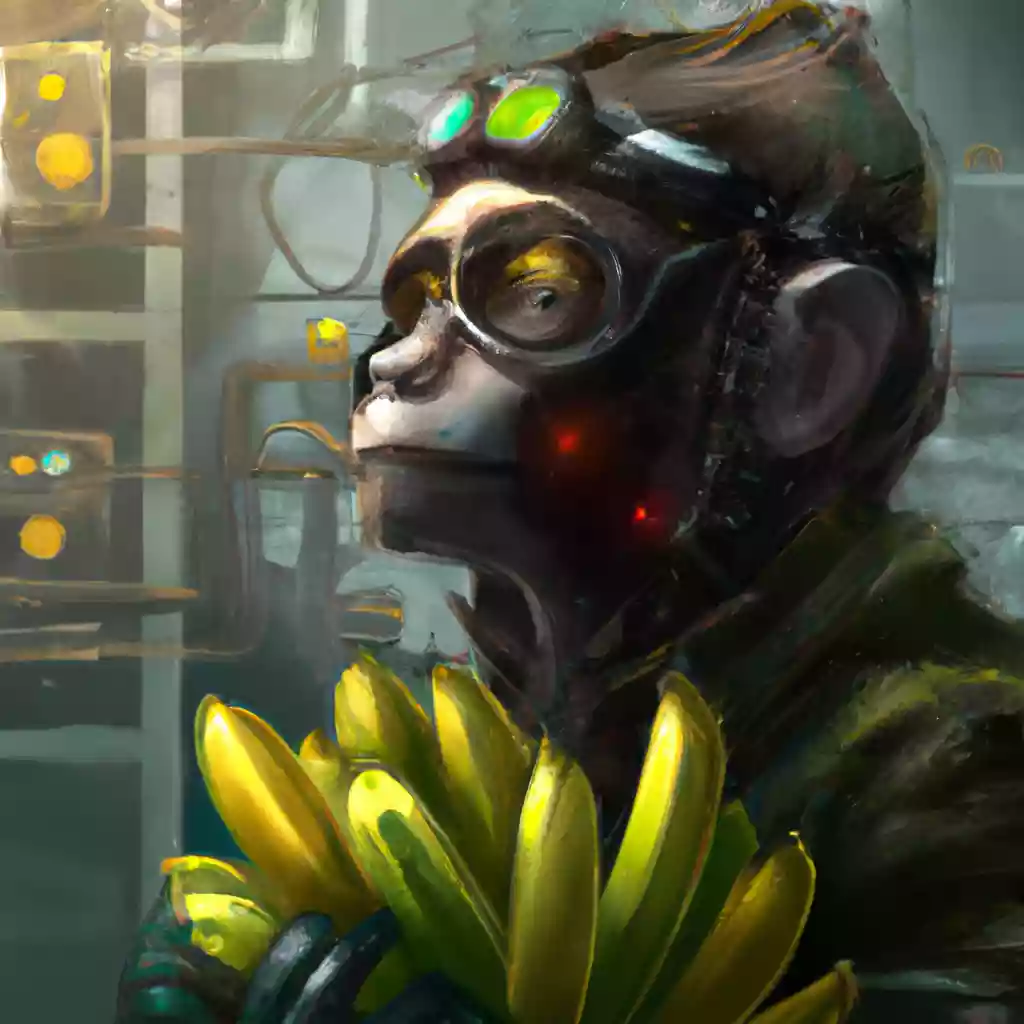
变化
变体端点生成类似于输入图像的新图像(变体)。
在这里,我们将生成上面生成的图像的变体。
所需输入:
- image (str):用作变体基础的图像。 必须是有效的 PNG 文件,小于 4MB,并且是方形的。
可选输入:
- n (int):要生成的图像数。 必须介于 1 和 10 之间。默认为 1。
- size (str):生成图像的大小。 必须是“256×256”、“512×512”或“1024×1024”之一。 较小的图像速度更快。 默认为“1024×1024”。
- response_format (str):返回生成图像的格式。 必须是“url”或“b64_json”之一。 默认为“网址”。
- user (str):代表您的最终用户的唯一标识符,这将有助于 OpenAI 监控和检测滥用行为。
# create variations # call the OpenAI API, using `create_variation` rather than `create` variation_response = openai.Image.create_variation( image=generated_image, # generated_image is the image generated above n=2, size="1024x1024", response_format="url", ) # print response print(variation_response)
{ "created": 1667611666, "data": [ { "url": "https://oaidalleapiprodscus.blob.core.windows.net/private/org-l89177bnhkme4a44292n5r3j/user-dS3DiwfhpogyYlat6i42W0QF/img-7HTTBl2k9l4Ir4BTHXnJvFz9.png?st=2022-11-05T00%3A27%3A46Z&se=2022-11-05T02%3A27%3A46Z&sp=r&sv=2021-08-06&sr=b&rscd=inline&rsct=image/png&skoid=6aaadede-4fb3-4698-a8f6-684d7786b067&sktid=a48cca56-e6da-484e-a814-9c849652bcb3&skt=2022-11-04T01%3A50%3A22Z&ske=2022-11-05T01%3A50%3A22Z&sks=b&skv=2021-08-06&sig=QlcKhn427bOAQobM8CmpEf3K90OiumP5jOQwkJpcH6Y%3D" }, { "url": "https://oaidalleapiprodscus.blob.core.windows.net/private/org-l89177bnhkme4a44292n5r3j/user-dS3DiwfhpogyYlat6i42W0QF/img-KGKrKGzlsXN0INxaeII2t8XG.png?st=2022-11-05T00%3A27%3A46Z&se=2022-11-05T02%3A27%3A46Z&sp=r&sv=2021-08-06&sr=b&rscd=inline&rsct=image/png&skoid=6aaadede-4fb3-4698-a8f6-684d7786b067&sktid=a48cca56-e6da-484e-a814-9c849652bcb3&skt=2022-11-04T01%3A50%3A22Z&ske=2022-11-05T01%3A50%3A22Z&sks=b&skv=2021-08-06&sig=RbPoAwXMVfdPxKF40ZjVjlclrnzaQZS%2BxzhgkEcYhOk%3D" } ] }
# save the images variation_urls = [datum["url"] for datum in variation_response["data"]] # extract URLs variation_images = [requests.get(url).content for url in variation_urls] # download images variation_image_names = [f"variation_image_{i}.png" for i in range(len(variation_images))] # create names variation_image_filepaths = [os.path.join(image_dir, name) for name in variation_image_names] # create filepaths for image, filepath in zip(variation_images, variation_image_filepaths): # loop through the variations with open(filepath, "wb") as image_file: # open the file image_file.write(image) # write the image to the file
# print the original image print(generated_image_filepath) display(Image.open(generated_image_filepath)) # print the new variations for variation_image_filepaths in variation_image_filepaths: print(variation_image_filepaths) display(Image.open(variation_image_filepaths))
./images/generated_image.png
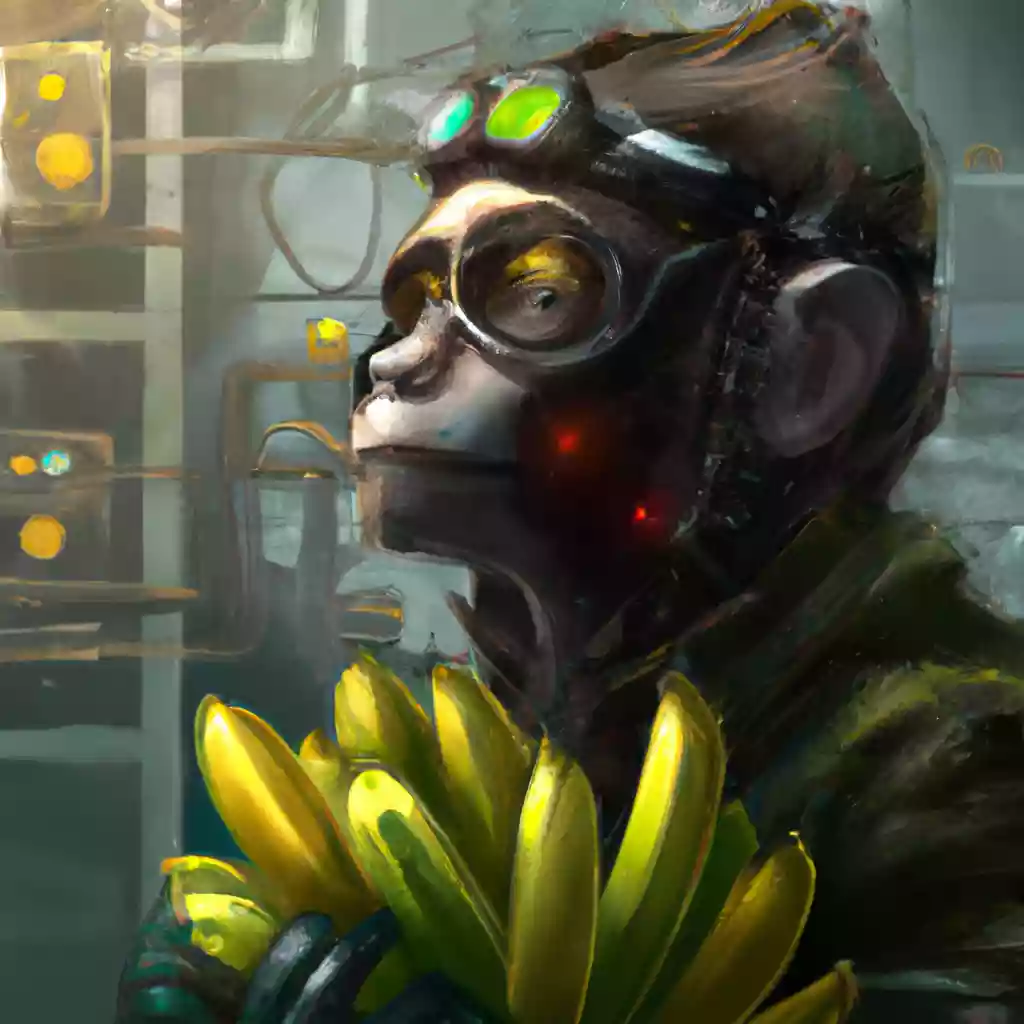
./images/variation_image_0.png
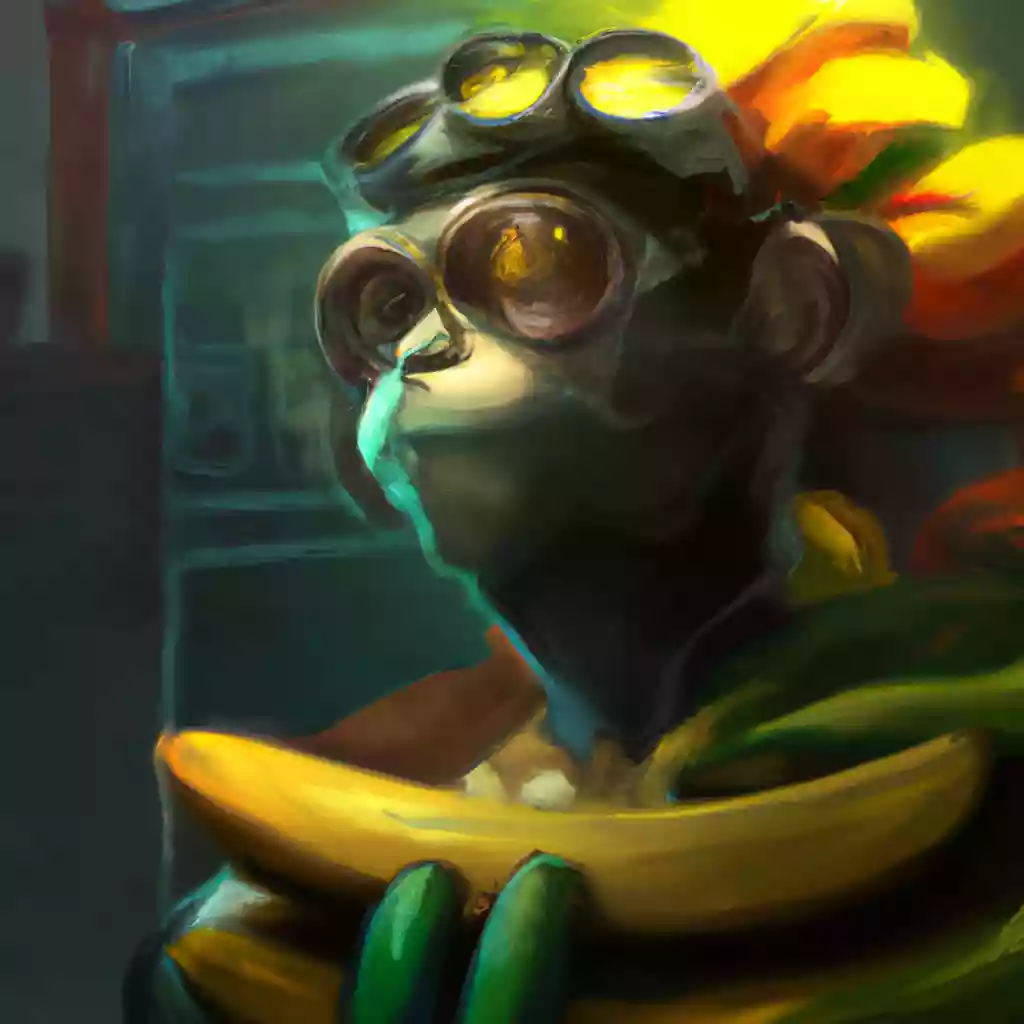
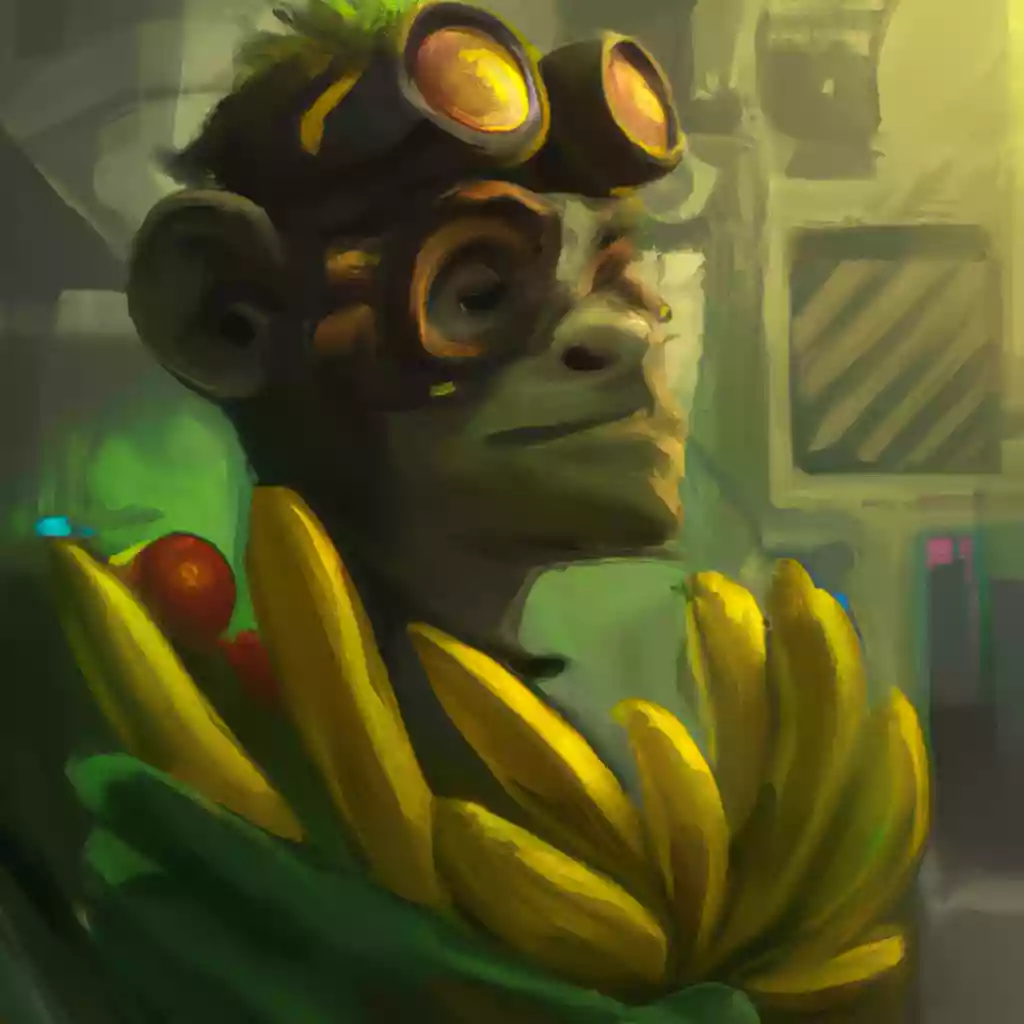
编辑
编辑端点使用 DALL-E 生成现有图像的指定部分。 需要三个输入:要编辑的图像、指定要重新生成的部分的遮罩以及描述所需图像的提示。
所需输入:
- image (str):要编辑的图像。 必须是有效的 PNG 文件,小于 4MB,并且是方形的。
- mask (str):附加图像,其完全透明区域(例如,alpha 为零的区域)指示应编辑图像的位置。 必须是有效的 PNG 文件,小于 4MB,并且与图像具有相同的尺寸。
- 提示(str):所需图像的文本描述。 最大长度为 1000 个字符。
可选输入:
- n (int):要生成的图像数。 必须介于 1 和 10 之间。默认为 1。
- size (str):生成图像的大小。 必须是“256×256”、“512×512”或“1024×1024”之一。 较小的图像速度更快。 默认为“1024×1024”。
- response_format (str):返回生成图像的格式。 必须是“url”或“b64_json”之一。 默认为“网址”。
- user (str):代表您的最终用户的唯一标识符,这将有助于 OpenAI 监控和检测滥用行为。 了解更多。
设置编辑区
编辑需要一个“mask”来指定要重新生成图像的哪一部分。 将重新生成 alpha 为 0(透明)的任何像素。 下面的代码创建了一个 1024×1024 的遮罩,其中下半部分是透明的。
# create a mask width = 1024 height = 1024 mask = Image.new("RGBA", (width, height), (0, 0, 0, 1)) # create an opaque image mask # set the bottom half to be transparent for x in range(width): for y in range(height // 2, height): # only loop over the bottom half of the mask # set alpha (A) to zero to turn pixel transparent alpha = 0 mask.putpixel((x, y), (0, 0, 0, alpha)) # save the mask mask_name = "bottom_half_mask.png" mask_filepath = os.path.join(image_dir, mask_name) mask.save(mask_filepath)
执行编辑
现在我们向 API 提供我们的图像、标题和蒙版,以获得 5 个图像编辑示例。
# edit an image # call the OpenAI API edit_response = openai.Image.create_edit( image=open(generated_image_filepath, "rb"), # from the generation section mask=open(mask_filepath, "rb"), # from right above prompt=prompt, # from the generation section n=1, size="1024x1024", response_format="url", ) # print response print(edit_response)
{ "created": 1667611683, "data": [ { "url": "https://oaidalleapiprodscus.blob.core.windows.net/private/org-l89177bnhkme4a44292n5r3j/user-dS3DiwfhpogyYlat6i42W0QF/img-F5XQFFBLrN7LdXuG5CkQJpxr.png?st=2022-11-05T00%3A28%3A03Z&se=2022-11-05T02%3A28%3A03Z&sp=r&sv=2021-08-06&sr=b&rscd=inline&rsct=image/png&skoid=6aaadede-4fb3-4698-a8f6-684d7786b067&sktid=a48cca56-e6da-484e-a814-9c849652bcb3&skt=2022-11-04T02%3A06%3A29Z&ske=2022-11-05T02%3A06%3A29Z&sks=b&skv=2021-08-06&sig=2UhH%2BkKdvDVoRcgWJhmNFVzpvLzBAZpnA/tU80Zc8M0%3D" } ] }
# save the image edited_image_name = "edited_image.png" # any name you like; the filetype should be .png edited_image_filepath = os.path.join(image_dir, edited_image_name) edited_image_url = edit_response["data"][0]["url"] # extract image URL from response edited_image = requests.get(edited_image_url).content # download the image with open(edited_image_filepath, "wb") as image_file: image_file.write(edited_image) # write the image to the file
# print the original image print(generated_image_filepath) display(Image.open(generated_image_filepath)) # print edited image print(edited_image_filepath) display(Image.open(edited_image_filepath))
./images/generated_image.png
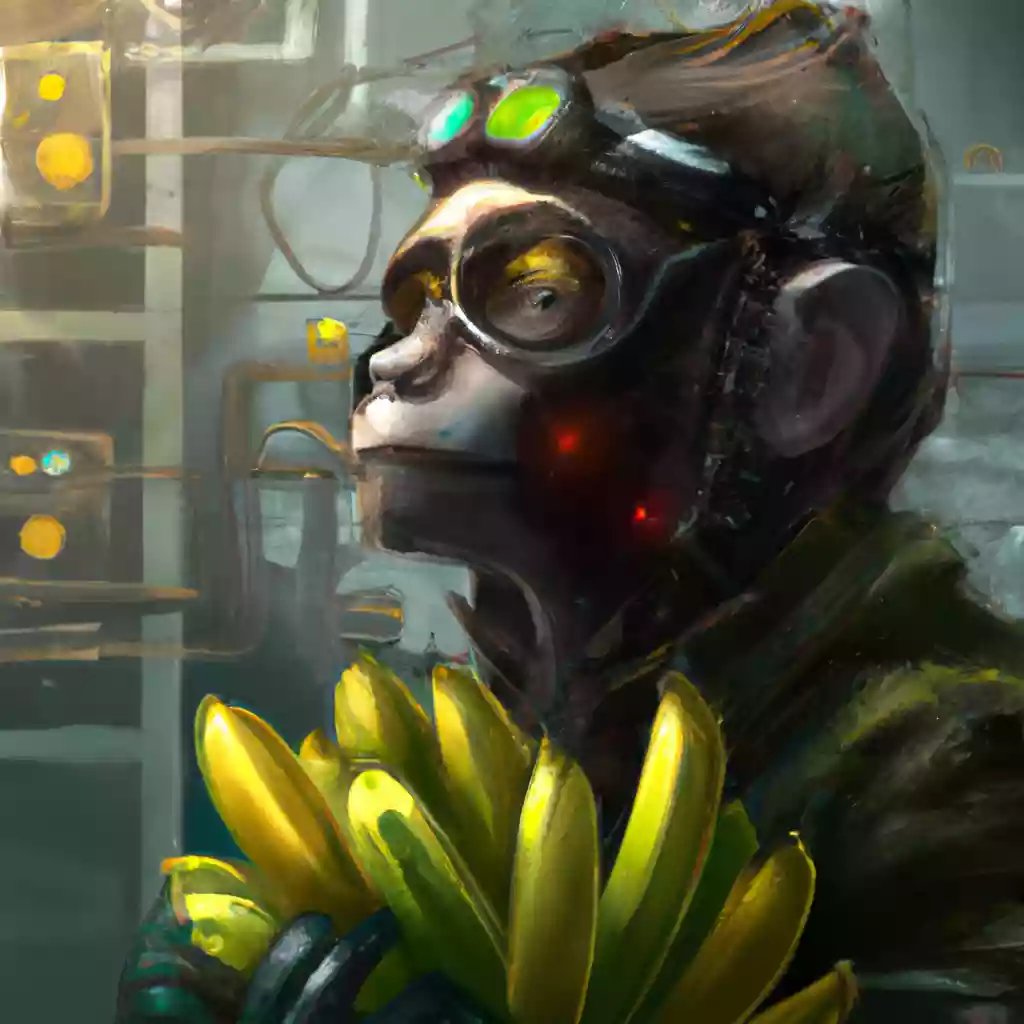
./images/edited_image.png
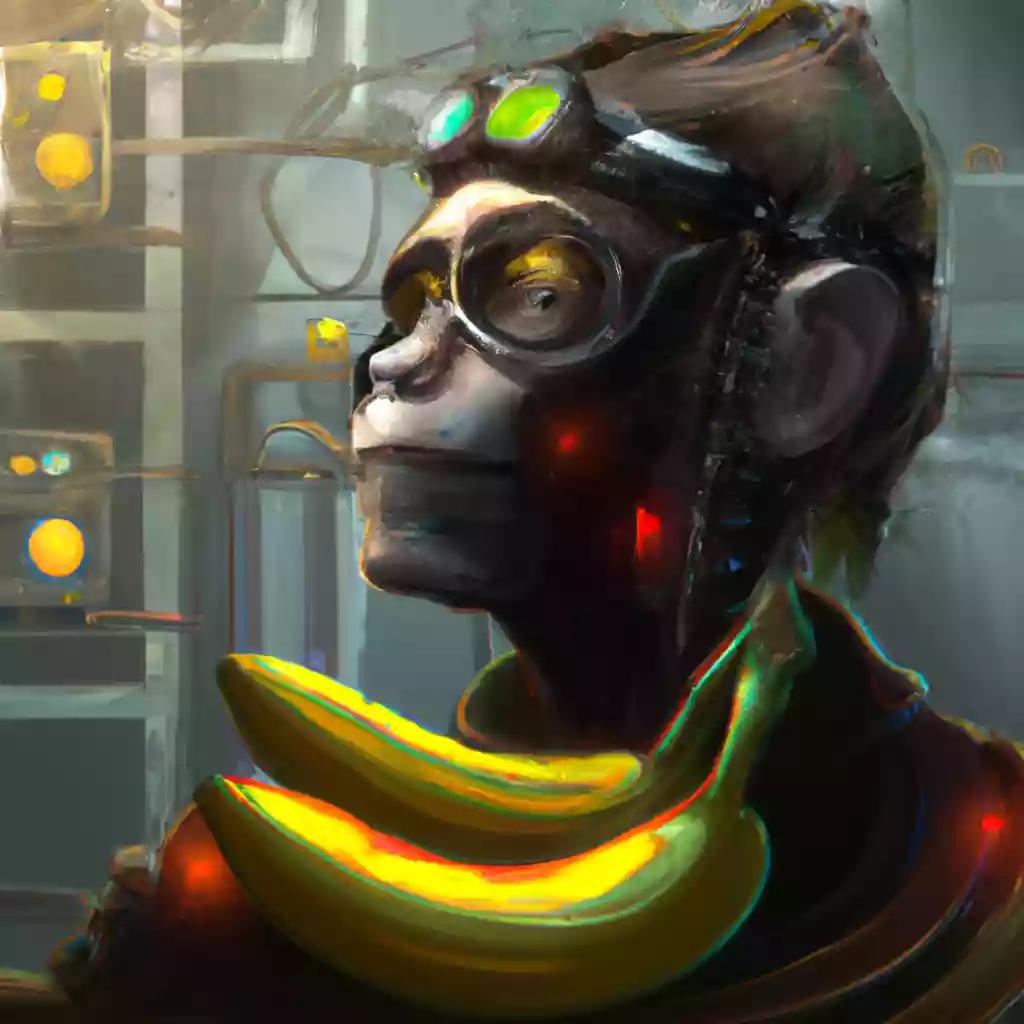
评论 (0)